We have talked about the DevOps Lifecycle and Mapping Tools previously. Under the Code stage of the lifecycle, the source control is the key component. And Git is the first word that jumps out. Remembering all the Git commands can be a daunting task. So we want to use this article to be the repository of common Git commands.
The diagram below demonstrate the Git workflow and the key commands. We’ll cover these key commands first, and then list out the other command options.
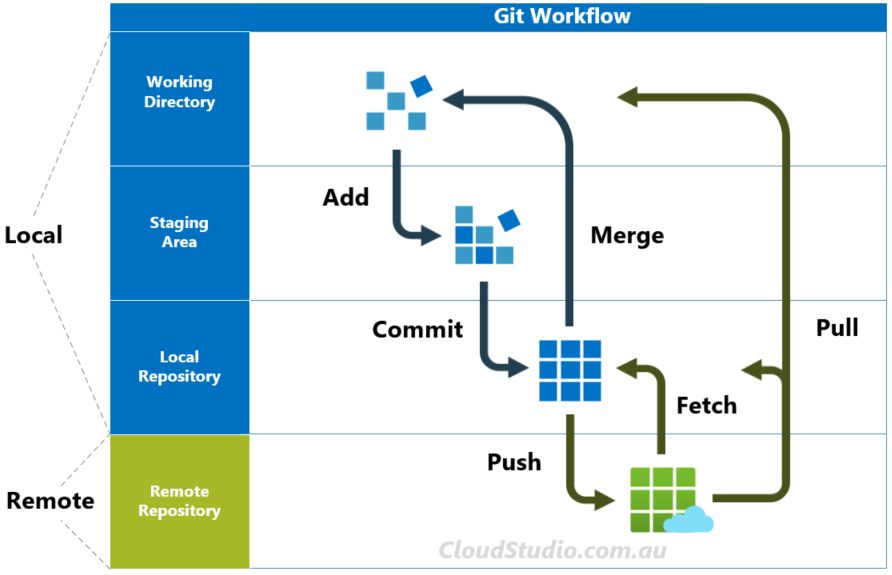
Please note that the workflow above is assuming that you’ve already established your local repository. If not, it’s ok. We’ll also include the initiation commands, like git init and git clone, in the Additional Commands section.
Key Commands
Commands | Description and Exmaples |
---|---|
git add | Stage changes for next commit git add . git add file_name git add c:\local_repo_name\repo_folder_name/sub_folder_name |
git commit | Commit the staged snapshot to the local repository git commit -m "commit message" |
git push | Upload local repository content to a remote repository git push origin master git push -u origin local_branch_name |
git fetch | Download content from remote repository, but doesn’t force the merge git fetch origin master |
git merge | Join two branches together git merge local_branch_name git merge local_branch_1 local_branch_2 |
git pull | Combo of git fetch and git merge git pull git pull origin remote_branch_name |
For detailed Git reference, see https://git-scm.com/docs
Additional Commands
To make it easier for searching, we’ve put the commands below in the alphabetical order.
Commands | Description and Examples |
---|---|
git blame | Review a file’s modification history git blame file_name git blame -L 1,10 file_name It’s often used together with git log to find out what, how and why a change was made to a file |
git branch | manage branches of a repository, e.g. create, list, rename and delete git branch new_branch_name git branch git branch -a git branch -m renamed_branch_name git branch -d existing_branch_name |
git checkout | Switch to another branch in the working directory git checkout local_branch_name git checkout -b new_branch_name |
git clean | Remove the untracked files git clean -n git clean -f git clean -dn git clean -df |
git clone | Clone a remote repository on to a local repository git clone https://github.com/aws/aws-toolkit-eclipse.git |
git config | Customise the git environment git config --list --show-origin git config --global user.name "Richard" git config user.name |
git diff | Inspect changes in a repository git diff git diff file_name git diff commit_id_1 commit_id_2 git diff branch_name_1 branch_name_2 |
git init | Create an empty Git repository in a specified directory git init c:\local_repo_name\repo_folder_name |
git log | View the commit history of a branch git log git log -p -3 |
git rebase | Move one branch to the tip of another branch git rebase base_branch_name *do not rebase any shared branches |
git reflog | View the HEAD change history of all local branches git reflog git reflog --all -3 |
git remote | Manage remote repositories’ information stored locally git remote -v git remote repository_name repository_url git remote rm repository_name |
git reset | Move both current HEAD pointer and branch ref pointer git reset --soft commit_id git reset --mixed commit_id git reset --hard commit_id git reset commit_id file_name |
git revert | Undo changes to a commit history git revert HEAD git revert commit_id git revert -n HEAD |
git rm | Remove tracked files from the staging area and (but not or) working directory git rm file_name_1 file_name_2 git rm --cached file_name_1 git rm -r folder_name |
git stash | Save and hide committed and uncommitted changes git stash save "stash_message" git stash -u git stash -a git stash -p git stash list git stash show git stash pop git clean -n -d -x |
git status | View the state of working directory and staging area git status git status -s |
git tag | Snapshot specific points in a repository history git tag git tag -a tag_name -m "tag message" git tag -a tag_name commit_id |
For detailed Git reference, see https://git-scm.com/docs
Advanced Tips
Case | Example |
---|---|
Set up git alias | git config --global alias.lol "log --oneline --graph --decorate" git config --global alias.ch "checkout" git config --global alias.br "branch" git config --global alias.co "commit" git config --global alias.st "status" |
Config levels | --local apply configuration at the repository level (<repository folder/.git/config>) --global apply configuration at the user level (C:\Users\<user name>\.gitconfig) --system apply configuration for all users across an entire machine (C:\ProgramData\Git\config) |
Customise git log format | git log --pretty=format:"%cn committed %h on %cd" git log --oneline --graph --decorate |
Filter git log output | git log --after="yyyy-mm-dd" --before="yyyy-mm-dd" git log --author="author1\|author2" git log --grep="commit_message_key_word" git log -S"file_content_key_word" git log branch_name_1 branch_name_2 |
Some plumbing commands | git cat-file -p HEAD git ls-tree -r HEAD git ls-files -s |
Wildcard git rm | git rm folder_name/\*.yml git rm *.tags |
Undo git rm | git reset HEAD git checkout . |
Glossary
Key Words | Explanation |
---|---|
origin | origin stands for the remote repository. When we use “git push -u origin local_branch_name“, it tells the system that we want to push our local branch to the remote repository. Usually there is one default remote repository and origin represents this default repository. If you don’t like this name, you can rename it by using “git remote rename origin new_name“ |
HEAD | HEAD stands for the last commit of the active/current branch. Each repository only has one current branch, hence one HEAD as well. If you want to check where the HEAD of a repository is pointing to, run “cat .git/HEAD“ Detached HEAD happens when a checkout command is applied to a specific historical commit, tag or remote branch. |
master | master is a branch, the default branch, the main branch, and it’s always there. |
branch | branch is a like a fork in the history of a repository. One branch represents an independent line of development, like a fork teeth. |
index | index is the proposed next commit, also called staging area. |
For detailed Git reference, see https://git-scm.com/docs
For undo scenarios, see https://github.blog/2015-06-08-how-to-undo-almost-anything-with-git/
For reset vs checkout, see https://git-scm.com/book/en/v2/Git-Tools-Reset-Demystified